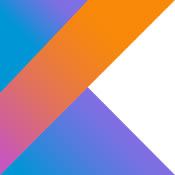
Suppose you wanted to print the string “Hello there!” three times. In any language that borrows its syntax from C, you’d probably use the classic for
loop, as shown below:
// C99
for (int i = 0; i < 3; i++) {
printf("Hello there!\n");
}
// JavaScript
for (let i = 0; i < 3; i++) {
console.log("Hello there!");
}
With Kotlin’s for
loop, you have a couple of options:
// Kotlin
// Here’s one way you can do it:
for (index in 0 until 3) {
println("Hello there!")
}
// Here’s another way:
for (i in 1..3) {
println("Hello there!")
}
If you’re used to programming in a C-style language, you’ll probably reach for a for
loop when you need to perform a task a given number of times. The Kotlin language designers noticed this and came up with something more concise: the repeat()
function:
repeat(3) {
println("Hello there!")
}
As you can see, using repeat()
give you a shorter, easier to read line than for
. And in most cases, shorter and easier to read is better.
Do you need to know the current iteration index? Here’s how you’d get that value:
fun main() {
repeat(3) { iteration ->
println("Hello there from iteration $iteration!")
}
}
Remember, you can use whatever variable name you want for that index, as long as it’s a valid name:
fun main() {
repeat(3) { thingy ->
println("Hello there from iteration $thingy!")
}
}
Note that repeat()
is for performing a task a known number of times, without exceptions. It’s a function that takes a closure, not a loop structure. This means that the break
and continue
statements won’t work:
// This WILL NOT compile!
fun main() {
repeat(3) { iteration ->
// 🚨🚨🚨 You can’t do this 🚨🚨🚨
if (iteration == 1) {
break
}
// 🚨🚨🚨 You can’t do this, either 🚨🚨🚨
if (iteration == 2) {
continue
}
// “break” and “continue” work only
// inside a loop, and repeat()
// *isn’t* a loop...
// ...it’s a *function*!
println("Hello there from iteration $iteration!")
}
}
For the curious, here’s the source code for repeat()
:
@kotlin.internal.InlineOnly
public inline fun repeat(times: Int, action: (Int) -> Unit) {
contract { callsInPlace(action) }
for (index in 0 until times) {
action(index)
}
}
To summarize: If you need to perform a task n times in Kotlin — and only n times — use repeat instead()
of for
!