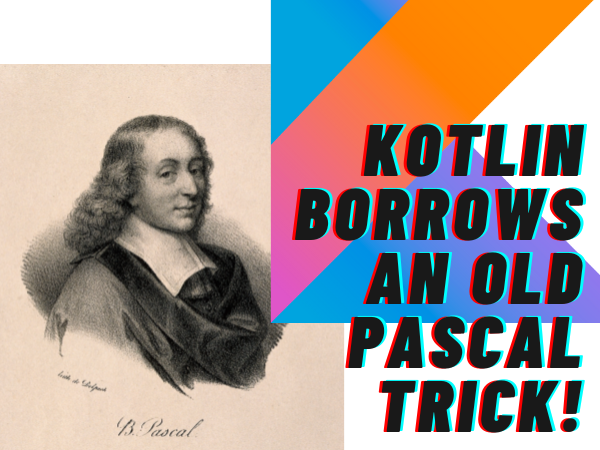
Suppose we have this Kotlin class:
// Kotlin
class ProgrammingLanguage(
var name: String,
var creator: String,
var yearFirstAppeared: Int,
var remarks: String,
var isInTiobeTop20: Boolean) {
fun display() {
println("${name} was created by ${creator} in ${yearFirstAppeared}.")
println("${remarks}")
if (isInTiobeTop20) {
println("This language is in the TIOBE Top 20.")
}
println()
}
}
Creating an instance is pretty straightforward:
// Kotlin
val currentLanguage = ProgrammingLanguage(
"Pascal",
"Nikalus Wirth",
1970,
"My first compiled and structured language. Also my first exposure to pointers.",
false)
When you run the code currentLanguage.display()
, you get:
Pascal was created by Nikalus Wirth in 1970. My first compiled and structured language. Also my first exposure to pointers. |
Suppose you want to change all the properties of currentLanguage
and then display the result. If you’re used to programming in other object-oriented programming languages, you’d probably do it like this:
// Kotlin
currentLanguage.name = "Miranda"
currentLanguage.creator = "David Turner"
currentLanguage.yearFirstAppeared = 1985
currentLanguage.remarks = "This was my intro to functional programming. It’s gone now, but its influence lives on in Haskell."
currentLanguage.isInTiobeTop20 = false
currentLanguage.display()
For the curious, here’s the output:
Miranda was created by David Turner in 1985. This was my intro to functional programming. It’s gone now, but its influence lives on in Haskell. |
Now, that’s a lot of currentLanguage
. You could argue that IDEs free us from a lot of repetitive typing, but it still leaves us with a lot of reading. There should be a way to make the code more concise, and luckily, the Kotlin standard library provides the with()
function.
The with()
function
One of the first programming languages I learned is Pascal. I cut my teeth on Apple Pascal on my Apple //e and a version of Waterloo Pascal for the ICON computers that the Ontario Ministry of Education seeded in Toronto schools.
Pascal has the with
statement, which made it easier to access the various properties of a record (Pascal’s version of a struct) or in later, object-oriented versions of Pascal, an object.
Kotlin borrowed this trick and implemented it as a standard library function. Here’s an example, where I change currentLanguage so that it contains information about BASIC:
// Kotlin
with(currentLanguage) {
name = "BASIC"
creator = "John Kemeny and Thomas Kurtz"
yearFirstAppeared = 1964
remarks = "My first programming language. It came built-in on a lot of ‘home computers’ in the 1980s."
isInTiobeTop20 = false
}
This time, when you run the code currentLanguage.display()
, you get…
BASIC was created by John Kemeny and Thomas Kurtz in 1964. My first programming language. It came built-in on a lot of ‘home computers’ in the 1980s. |
…and the code is also a little easier to read without all those repetitions of currentLanguage
.
with()
is part of the Kotlin standard library. It has an all-too-brief writeup in the official docs, and as a standard library function, you can see its code.