(In case you missed it, here’s the previous article in this series.)
FizzBuzz became popular in the late 2000 – 2010 decade, which was around the same time that may programmers were beginning to rediscover functional programming. I say rediscover rather than discover because functional programming goes back all the way to Lisp, whose spec was written in 1958, which is the dawn of time as far as modern computing is concerned. As Wikipedia puts it, Lisp is “the second-oldest high-level programming language in widespread use today. Only Fortran is older, by one year.”
(Both Fortran and Lisp are heavily based in mathematics — in fact, Fortran is short for FORmula TRANslation. This is one of the reasons that there’s a strong math bias in programming to this day.)
One senior developer I know tested prospective developers’ functional programming skills by issuing this test to anyone who passed the original FizzBuzz test:
Write FizzBuzz, but this time, instead of FizzBuzzifying the numbers 1 through 100, FizzBuzzify the contents of an array, which can contain any number of integers, in any order.
(The senior developer didn’t use the word “FizzBuzzify,” but I think you get my point.)
The resulting app, if given this array…
[30, 41, 8, 26, 3, 7, 11, 5]
…should output this array:
[‘FizzBuzz’, 41, 8, 26, ‘Fizz’, 7, 11, ‘Buzz’]
Note that the original array contained all integers, while the result array can contain both strings and integers. The senior developer was interviewing programmers who’d be working in Ruby, where you can easily use arrays of mixed types.
You’d get a passing grade if your solution simply adapted the original FizzBuzz to take an array as its input. Here’s a Python implementation of that solution:
def fizzBuzz_list_imperatively(numbers): finalResult = [] for number in numbers: currentResult = None isMultipleOf3 = (number % 3 == 0) isMultipleOf5 = (number % 5 == 0) if isMultipleOf3 and isMultipleOf5: currentResult = "FizzBuzz" elif isMultipleOf3: currentResult = "Fizz" elif isMultipleOf5: currentResult = "Buzz" else: currentResult = number finalResult.append(currentResult) return finalResult
However, the developer was looking for a more functional approach. In functional programming, if you’re being asked to perform some kind of calculation based on the contents of a list, you should probably use a map, filter, or reduce operation.
In case you’re not quite familiar with what these are, here’s a simple explanation that uses emojis:
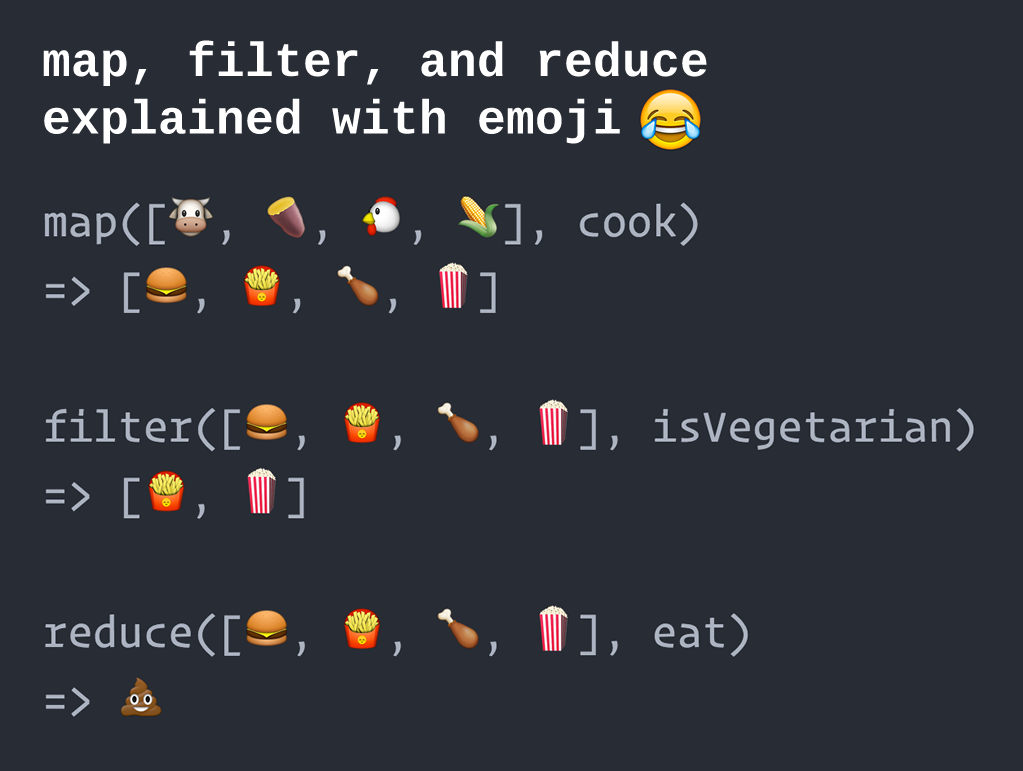
The map operation, given a list and a function, applies that function to every item in the given list, which creates a new list. The senior developer granted bonus points to anyone who came up with a map-based solution.
Here’s a Python implementation of what the senior developer was looking for:
def fizzBuzz_list_functionally(number_list): return list(map(fizzBuzzify, number_list)) def fizzBuzzify(number): isMultipleOf3 = (number % 3 == 0) isMultipleOf5 = (number % 5 == 0) if isMultipleOf3 and isMultipleOf5: return "FizzBuzz" elif isMultipleOf3: return "Fizz" elif isMultipleOf5: return "Buzz" else: return number
This implementation breaks the problem into two functions:
- A
fizzBuzzify()
function, which given a number, returns Fizz, Buzz, FizzBuzz, or the original number, depending on its value, and - A
map()
function, which appliesfizzBuzzify()
across the entire array.
Remember, the senior developer was looking for Ruby developers, and Ruby doesn’t support nested functions. Python does, however, and I like packaging things neatly to prevent errors. I think that if a function a makes exclusive use of another function b, you should nest b inside a.
With that in mind, let’s update fizzBuzz_list_functionally()
:
def fizzBuzz_list_functionally(number_list): def fizzBuzzify(number): isMultipleOf3 = (number % 3 == 0) isMultipleOf5 = (number % 5 == 0) if isMultipleOf3 and isMultipleOf5: return "FizzBuzz" elif isMultipleOf3: return "Fizz" elif isMultipleOf5: return "Buzz" else: return number return list(map(fizzBuzzify, number_list))
What’s next
In the next installment in this series, we’ll look at FizzBuzz solutions that don’t use the modulo (%
) operator.
Previously, in the “Programmer interview challenge” series
- Programmer interview challenge 1: Anagram
- Programmer interview challenge 1, revisited: Revising “Anagram” in Python and implementing it in JavaScript
- Programmer interview challenge 1, revisited again: “Anagram” in Swift, the easy and hardcore way
- Programmer interview challenge 1, revisited once more: “Anagram” in Ruby and C#
- Programmer interview challenge 2: The dreaded FizzBuzz, in Python