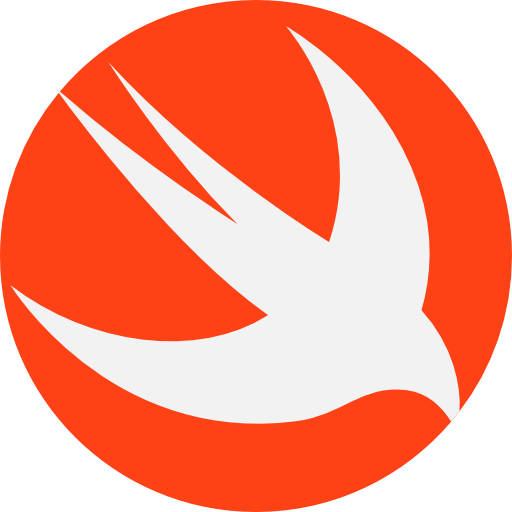
Earlier this week, I posted the first article in the How to solve coding interview questions series: Find the first recurring character in a string.
Here’s the challenge:
Write a Python function named
first_recurring_character()
or a JavaScript function namedfirstRecurringCharacter()
that takes a string and returns either:° The first recurring character in the given string, if one exists, or
° A null value like JavaScript’s or Kotlin’s
null
, Python’sNone
, or Swift’snil
.
Here’s the Swift version of my solution:
// Swift
func firstRecurringCharacter(text: String) -> String? {
var previouslyEncounteredCharacters = Set<Character>()
for character in text {
if previouslyEncounteredCharacters.contains(character) {
return String(character)
} else {
previouslyEncounteredCharacters.insert(character)
}
}
return nil
}
The Swift implementation differs from the Python and JavaScript versions due to Swift’s stricter typing
- Swift’s
Set
requires you to specify the type of things that it will store. In this case, we want to store items of typeCharacter
. - When looping through the items in a string with a
for
loop in Swift, you getCharacter
items. That’s whypreviouslyEncounteredCharacters
storesCharacter
items and notString
items. Once the function detects the first recurring character, it converts that character into a string and returns that value.