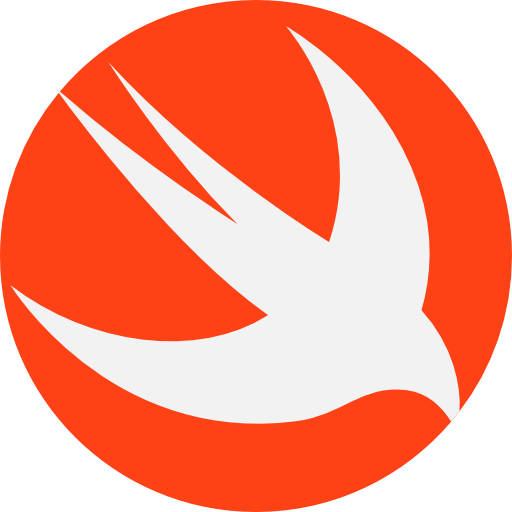
Converting a number into its word form — for example, converting 1,234,456 into one million, two hundred and thirty-four thousand, four hundred and fifty-six — used to be a really painful exercise in programming. These days, there are libraries that save you from having to do this, and in the case of Swift, this functionality is built into its standard library in the form of the NumberFormatter
class. Not only will it convert numbers into words, but it will also do so for many languages!
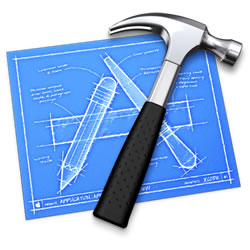
Open a Swift playground in Xcode and enter the following code:
// Swift
let formatter = NumberFormatter()
formatter.numberStyle = .spellOut
let number = 87654
let spelledOutNumber = formatter.string(for: number)!
print("\(number) spelled out is \(spelledOutNumber).")
Run the playground code, and you’ll see this:
87654 spelled out is eighty-seven thousand six hundred fifty-four. |
Having come from the world of C, where you format strings using printf()
and formatting strings, and later from other languages where you use whatever formatting method its string class provides, I’ve ignored most of Swift’s classes that derive from Formatter
— with one notable exception: DateFormatter
, which is indispensable when working with dates and times.
I’m now looking for an excuse to use this capability.

As I typed “DateFormatter” a couple of paragraphs above, I remembered that DateFormatter
had a locale
property. It’s for ensuring that any dates you present are in the correct form for the locale:
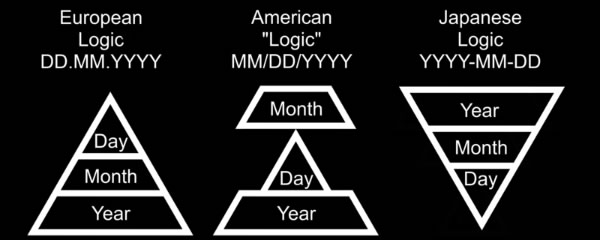
I wondered:
- Does
NumberFormatter
have alocale
property? - What happens if I changed it to something other than my system’s default of US English?
So I changed the code in my playground to the following:
// Swift
let formatter = NumberFormatter()
formatter.numberStyle = .spellOut
formatter.locale = Locale(identifier: "fil_PH")
let number = 87654
let spelledOutNumber = formatter.string(for: number)!
print("\(number) spelled out in Filipino is \(spelledOutNumber).")
I ran the code and saw this…
87654 spelled out in Filipino is walóng pû’t pitóng libó’t anim na daán at limáng pû’t ápat. |
…and my response was “Ay nako!” (translation: OMG!)
How about Korean?
// Swift
let formatter = NumberFormatter()
formatter.numberStyle = .spellOut
formatter.locale = Locale(identifier: "ko_KR")
let number = 87654
let spelledOutNumber = formatter.string(for: number)!
print("\(number) spelled out in Korean is \(spelledOutNumber).")
The output:
87654 spelled out in Korean is 팔만 칠천육백오십사. |
My response: 세상에 (“Sesange!”, which is pretty much Korean for OMG!)
Try it out! You might find this list of iOS locale string identifiers useful.

Speaking of Swift development, I’m restarting the Tampa Bay Apple Coding Meetup in the next few weeks. Watch this space, and if you’re interested, join the meetup group!
One reply on “Convert a number into words in a couple of lines of Swift”
[…] If you’re an iOS/macOS programmer, you’ll want to look at this article from a couple of days ago, Convert a number into words in a couple of lines of Swift. […]