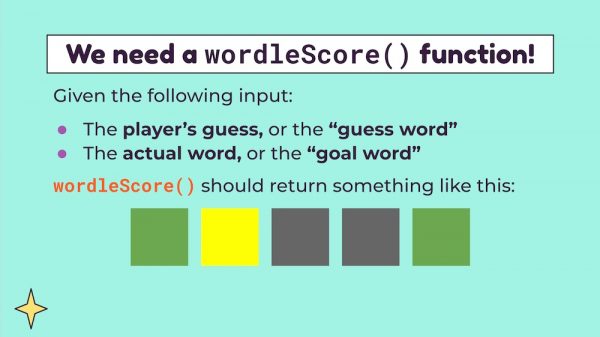
These slides capture what we worked on Tuesday night’s “Think Like a Coder” meetup: coming up with a first attempt at a “Wordle” function. Given a guess word and a goal word, it should output a Wordle-style score.

We came up with a solution that I like to call the “close enough” algorithm. It goes through the guess word one character at a time, comparing the current guess word character with the goal word character in the same position.
When making those character-by-character comparisons, the function follows the rules:
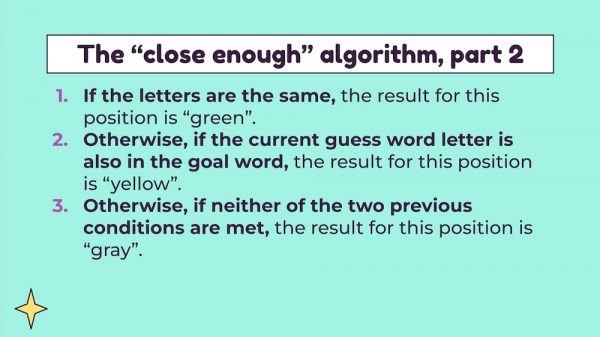
Here’s the “close enough” algorithm, implemented in Python…
def wordle(guess_word, goal_word):
# Go through the guess word one character at a time, getting...
#
# 1. index: The position of the character
# 2. character: The character at that position
for index, character in enumerate(guess_word):
# Compare the current character in the guess word
# to the goal word character at the same position
if character == goal_word[index]:
# Current character in the guess word
# matches its counterpart in the goal word
print("green")
elif character in goal_word:
# Current character in the guess word
# DOESN’T match its counterpart in the goal word,
# but DOES appear in the goal word
print("yellow")
else:
# Current character DOESN’T appear in the goal word
print("gray")
…and here’s the JavaScript implementation:
function wordle(guessWord, goalWord) {
// Go through the guess word one character at a time
for (let index in guessWord) {
// Compare the current character in the guess word
// to the goal word character at the same position
if (guessWord[index] == goalWord[index]) {
// Current character in the guess word
// matches its counterpart in the goal word
console.log('green')
} else if (goalWord.includes(guessWord[index])) {
// Current character in the guess word
// DOESN’T match its counterpart in the goal word,
// but DOES appear in the goal word
console.log('yellow')
} else {
// Current character DOESN’T appear in the goal word
console.log('gray')
}
}
}
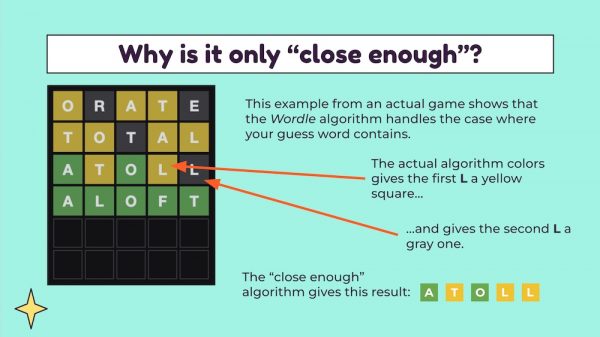
I call the solution “close enough” because yellow is a special case in Wordle. If the guess word is ATOLL and the goal word is ALOFT, the first L in ATOLL should be yellow and the second should be gray because there’s only one L in ALOFT.
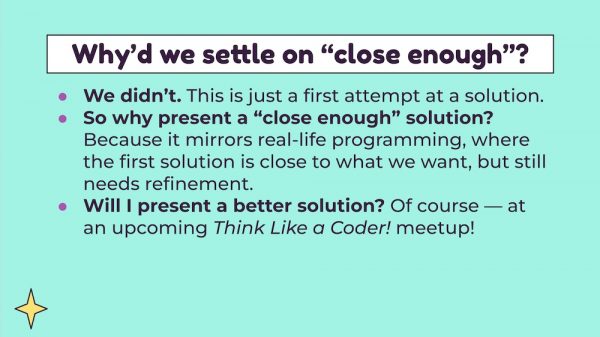
We didn’t settle on the “close enough” algorithm — it was just enough for that night’s session. In the next session, we’ll refine the algorithm so that it matches Wordle’s!

Want to become a better programmer? Join us at the next Think Like a Coder meetup!